You might see every method to insert JSON data into MySQL using PHP. So, we explain about insert multiple JSON data with an example of code in PHP.
Here, we implement all points step by step using PHP. Also, you can see how to store JSON data in the MySQL database with an example.
<html>
<head>
<title>Insert JSON Data</title>
</head>
<body>
<?php
$josndata = '{
"student":{ "name":"Harry", "country":"United State", "ContactNo":2545454 }
}';
// Database connection
$conn = new mysqli('localhost', 'username', 'password', 'databasename');
// Insert data Query
$sql = "INSERT INTO student_table ( name, jsondata )
VALUES ('Harry', '$josndata')";
if ($conn->query($sql) === TRUE) {
echo "Insert your JSON record successfully";
}
?>
</body>
</html>
Table of Contents
How to Insert JSON data into MySQL using PHP
In these articles, we have mentioned the code of store JSON data in MySQL using PHP. Now, we explain some steps to clarify all points to the above code.
Step 1: Firstly, you have to JSON data store a PHP variable. Also, have to store multiple JSON data as you want.
As well as, you can do from JSON file after JSON file read and get the data as a variable in PHP.
$josndata = '{
"student":{ "name":"Rohan", "country":"India", "Contact":25487545}
}';
Here, you saw a JSON data above section to store a variable. So, you have to create many types of JSON data from the array and other ways.
Thus, JSON can do read multiple data and insert JSON data into a single MySQL database table.
Step 2: After that, you create a Php code for Store JSON data. Firstly, create a database connection using PHP.
$servername = "localhost";
$username = "root";
$password = "";
$dbname = "student";
// Database connection
$conn = new mysqli($servername, $username, $password, $dbname);
So, the database provides a custom code for connection. Therefore, there some terms to mandatory to access database server name, username, password, and database name.
Step 3: Thus, we have to create an SQL query to insert JSON data into a MySQL database using Php. Also, we provide a store query for JSON data.
$sql = "INSERT INTO student_table ( fieldName1, FieldName2 )
VALUES ('Harry', '$jsondata-variable')";
So, in this code you can see there is a custom insert query in which provided by SQL. Afterward, showing in this query table name.
it is mandatory which table you have to provide for store JSON data.
Also, this SQL query has some fields which show inserting your data as a database field.
OUTPUT:
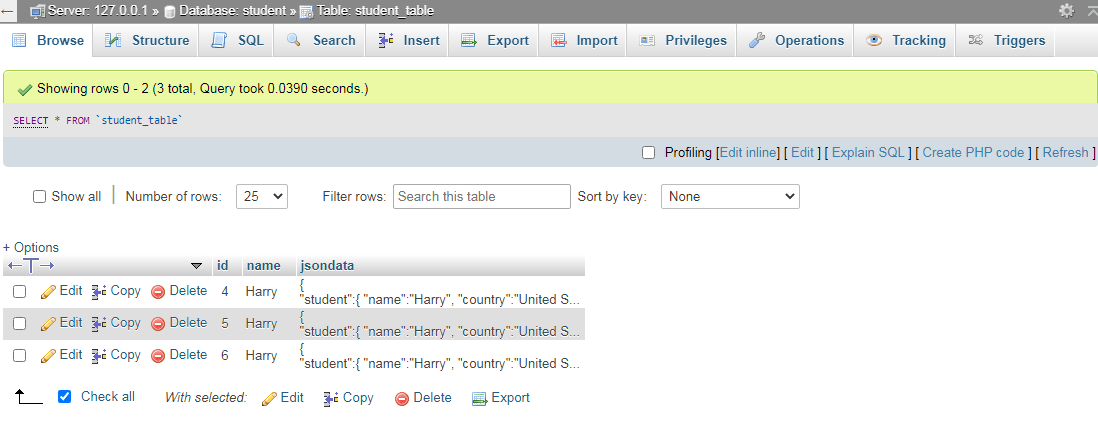
Store JSON data in MySQL Database
In this paragraph, we have other ways to store JSON data in the MySQL database.
So, as you know if you want to insert an only a single item from JSON data.
<html>
<head>
<title>Store JSON Data</title>
</head>
<body>
<?php
$josndata = '{
"student":{ "name":"Harry", "country":"United State", "ContactNo":2545454 }
}';
$data = json_decode($josndata);
$singledata = $data->student->name;
$servername = "localhost";
$username = "root";
$password = "";
$dbname = "student";
// Database connection
$conn = new mysqli($servername, $username, $password, $dbname);
$sql = "INSERT INTO student_table ( name, jsondata )
VALUES ('Harry', '$singledata')";
if ($conn->query($sql) === TRUE) {
echo "Store data only single successfully";
}
?>
</body>
</html>
OUTPUT:
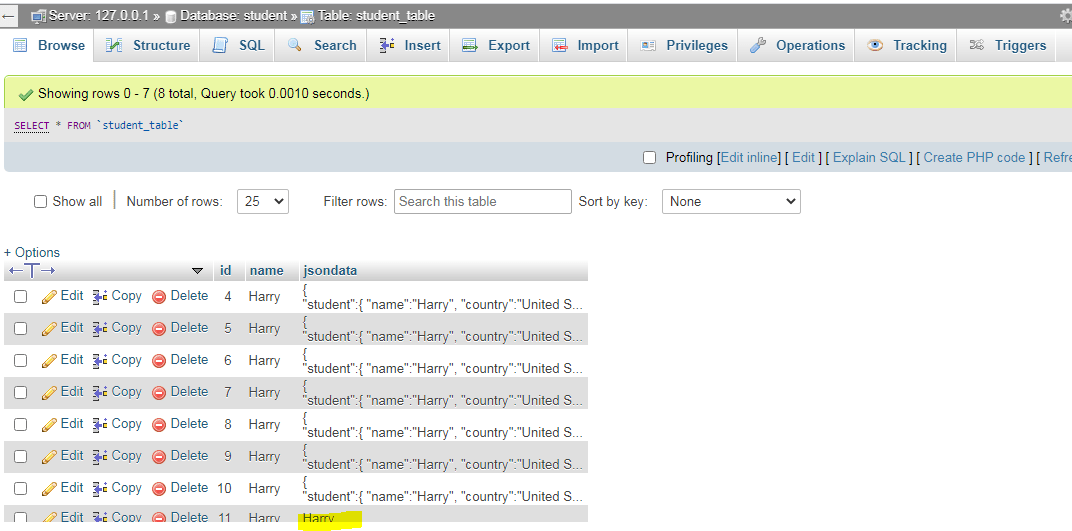
As you saw above code of store JSON data in MySQL in Php for single item values is stored from JSON.
Here, we stating the JSON data store a variable whole over data the variable name $josndata.
After that, convert JSON data to array $data = json_decode($josndata); then specific array value getting a variable $singledata = $data->student->name; this is two variable.
As a rule, we can do any method to store array value in the MySQL database using Php. Also, check these articles SQL Insert Multiple Rows.
Likewise, we have to use a SQL query all the above code section but they don’t have condition only simple query has used.
Now, I am going to start some other methods to implement in your Php code for inserting data with example.
Similarly, if you have JSON data then might be converted arrays using json_encode() function.
As well as, when gets the array of data might be put data everywhere. Also, insert data with the SQL query as you want.
How to Insert Multiple JSON Data into MySQL Database in Php
Similarly, we have to use the above section code the same as applying to insert multiple JSON data into the MySQL database in Php.
- firstly, created a Multi JSON file and write the file by the PHP code.
- After that, access the file a Php variable.
- Create a database connection using MySQL database syntax.
- Then add an Insert SQL query to store data in which holds on the variable.
- Finally, showing your result as you want on the front and screen.
Note: the main SQL query to insert JSON to MySQL in Php only holds single data which store at one time. After that, you can store multi times.
These are some points following when you program code. As well as, we have an example for multiple JSON data.
<html>
<head>
<title>Insert Multiple JSON Data</title>
</head>
<body>
<?php
$josndata = '{
"Customer":{ "name":"Jonny", "country":"CANADA", "ContactNo":524587777 },
"Student":{ "name":"Gussy", "country":"USA", "ContactNo":9856452100}
}';
// Database connection
$conn = new mysqli('localhost', 'username', 'password', 'databasename');
// Insert data Query
$sql = "INSERT INTO student_table ( name, jsondata )
VALUES ('Multidata', '$josndata')";
if ($conn->query($sql) === TRUE) {
echo "Insert your Multiple JSON record successfully";
}
?>
</body>
</html>
OUTPUT:

Afterward, you saw this example with all steps by step explained. Now, there have 2 records in the JSON data if you have more data you can store multiple data the same as the above code.
As wll as, create conditions in over code and query.
Conclusion
Finally, we discuss all possible methods about insert JSON data into MySQL using PHP. So, if you and douts in this method and functionality ask us to drop your queries with us.
Similarly, Learn the method of Php to other important topics Array to String Conversion in PHP.
Best tutorial
the best solution I need more
Sure i will provide soon
This really answered my problem, thank you!